Use TailwindCSS class utilities in React Native

Victor Bruce
Tue May 28 2024
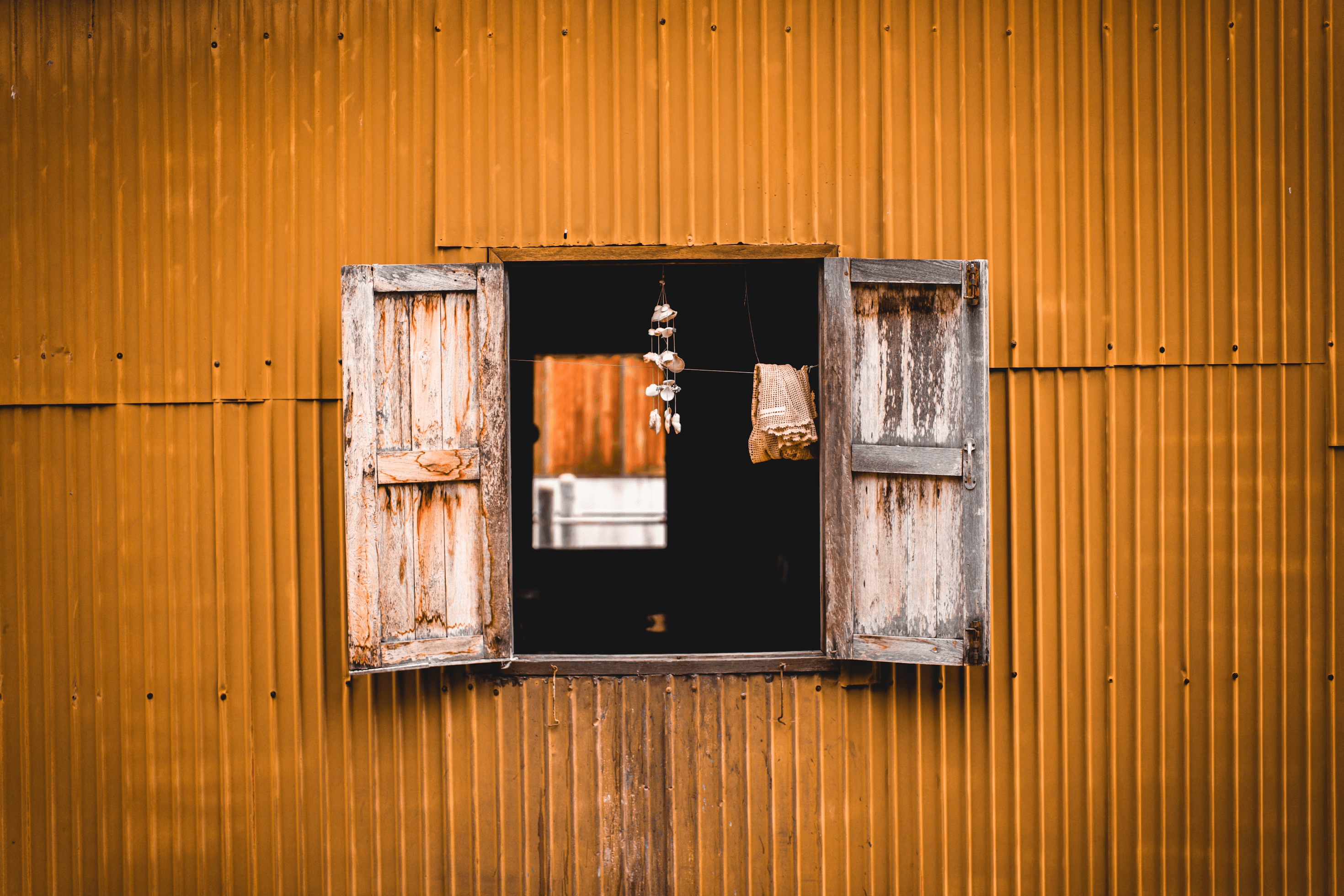
Styling a React Native App
Traditionally, React Native provides us with the StyleSheet object(an abstraction similar to CSS StyleSheets) where we write CSS styles to style our React Native app.
import {StyleSheet, View, Text} from "react-native"
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.title}>React Native</Text>
</View>
)
}
const styles = StyleSheet.create({
container: {
flex: 1,
padding: 24,
background: "#eaeaea"
},
title {
fontSize: 30,
fontWeight: "bold"
}
})
As of 2024, one of the favorite ways for most developers to style their web applications is by using Tailwind CSS. Tailwind CSS is a styling library that provides a series of classes that can be composed to build any design, directly in your code.
On mobile, NativeWind provides that compatibility layer between React Native and CSS. You can now transfer the skills you have in writing CSS using Tailwind on the Web, right in your React Native code.
Steps:
1. Installation
// NPM
npm install nativewind
npm install --save-dev tailwindcss@3.3.2
// YARN
yarn add nativewind
yarn add --dev tailwindcss@3.3.2
2. Setup Tailwind CSS
Run npx tailwindcss init to create a tailwind.config.js file. After, add the path to all of your component files in your `tailwind.config.js` file to apply the necessary styling. This step is crucial and missing it will cause you not to see the CSS classes applied.
// tailwind.config.js
module.exports = {
- content: [],
+ content: ["./App.{js,jsx,ts,tsx}", "./<custom directory>/**/*.{js,jsx,ts,tsx}"],
theme: {
extend: {},
},
plugins: [],
}
3. Add the Babel plugin
Inside your React Native project, modify the `babel.config.js` file by adding the line `plugins: ["nativewind/babel"]`
// babel.config.js
module.exports = function (api) {
ap.cache(true);
return {
presets: ["babel-preset-expo"],
+ plugins: ["nativewind/babel"],
};
};
Start writing Code.
Finally, you can add your TailwindCss classes you love to write!
import {Text, View} from "react-native"
export default function Index() {
return (
<View className="flex-1 items-center justify-center">
<Text className="text-2xl text-blue-400">Hello World!</Text>
</View>
)
}
Typescript Issue
If you're using Typescript in your React Native project, you will notice a squiggle line under the `className`. To solve this issue, create a type declaration file let's say `nativewind-env.d.ts` and add the following
/// <reference types="nativewind/types" />