Setting up Google Analytics in Your NextJs Project

Victor Bruce
Mon Jun 27 2022
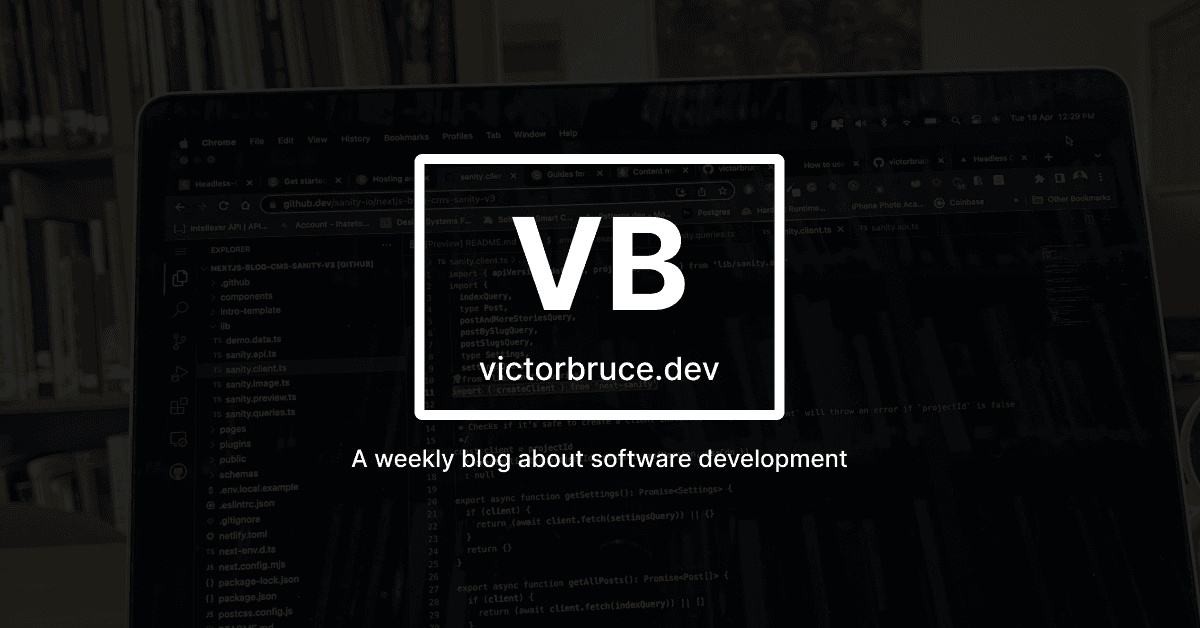
Analytics is a way to gather metrics about your business in an eye-opening experience. Knowing where your users come from, events users trigger on your web pages, the number of users that return to your site(retention), or the pages and features your users interact with can go a long way to help you build a successful product.
I wrote this blog for my future self. Navigating through documentation and other resources can take a lot of time. Also, it took me a long time to understand the features provided by Google Analytics so that I could take full advantage of the platform.
Blog Format
This blog will be in two parts. The first part will cover the technical aspect of setting up Google Analytics in your NextJs project.
The second part of this blog post will cover the questions bothering me whiles setting up Google Analytics, most of which I found answers to through research.
Part 1: Technical Aspect of Setting Up Google Analytics in NextJs
1. Visit analytics.google.com and sign in with your Google account
2. Navigate to the admin page by clicking on the admin link at the bottom left section of the side navigation bar.
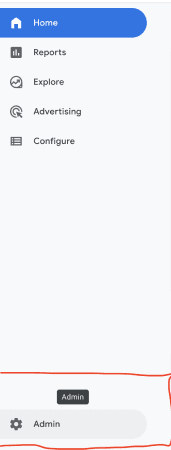
3. Click the create property button to create a new Google Analytics 4 property.
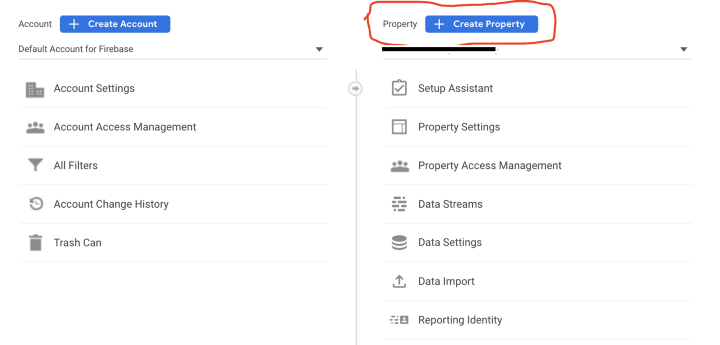
4. Provide a name for your property and fill in your business information to create a Property.
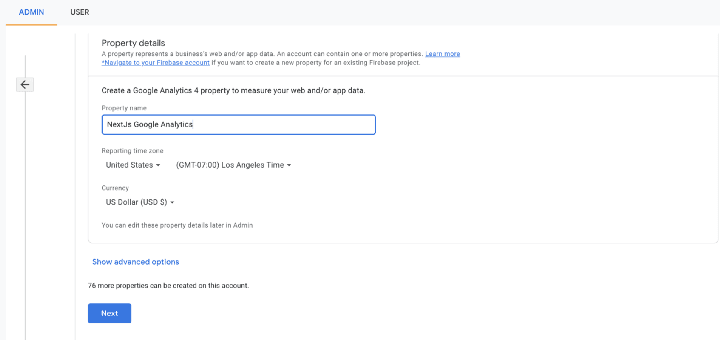
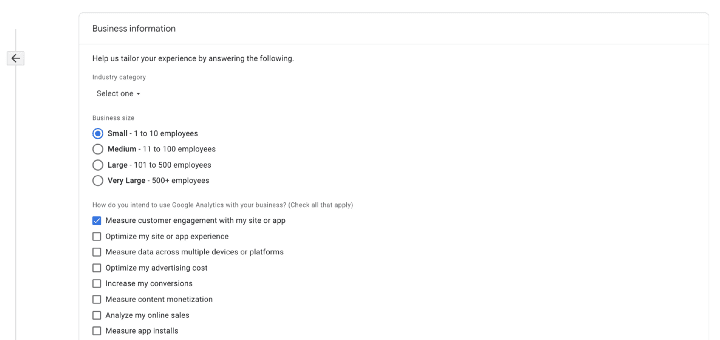
5. The next step is to set up a data stream to start collecting data. The data stream lets Google Analytics know where we will be gathering the data from(web, android app, or iOS app). In our case, we will choose the web option. Now, provide all the necessary details.
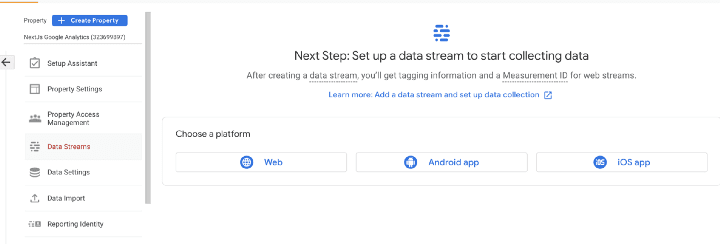
6. Finally, collapse the Global site tag(gtag.js) section and copy the code in a safe place. It will come in handy very soon.
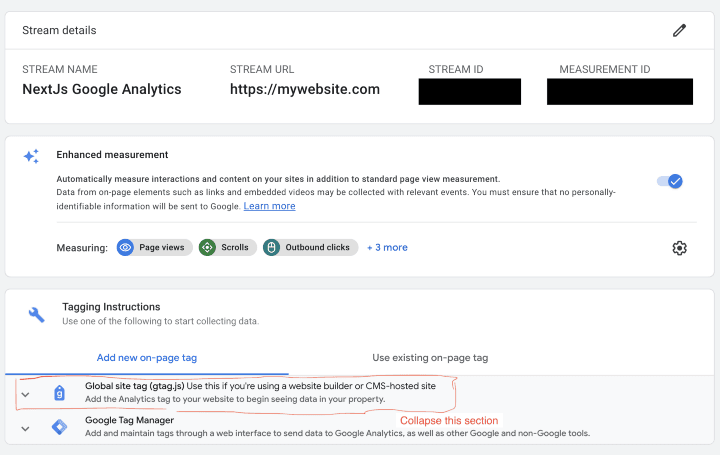
At this point, you have your measurement ID and Global site tag(gtag.js) code. Let’s jump into adding Google Analytics to our NextJs project in the next section.
Adding Google Analytics Global Site Tag(gtag.js) in our NextJs Project
One thing about analytics is that it should be running on every page. In NextJs, any shared layout between all pages is moved to a custom _app.js file. Hence, we will inject the gtag script code within this file so that we get data from each page.
But first, let's create some reusable functions. One for getting page views, and the other for getting events such as button clicks, video clicks, etc.
// exporting our google analytics measurement id from our .env file
export const GA_TRACKING_ID = process.env.NEXT_PUBLIC_GOOGLE_ANALYTICS
// records data on each page visited
export const pageView = (url: string) => {
window.gtag('config', GA_TRACKING_ID, {
page_path: url
})
}
// handles any custom event we want to track. For eg. how many clicks has our subscribe button
export const event = ({action, category, label, value}: any) => {
window.gtag('event', action, {
event_category: category,
event_label: label,
value: value
})
}
Secondly, let’s add the gtag script code in our __apps.js
file.
import {useEffect} from 'react'
import {useRouter} from 'next/router'
import type { AppProps } from 'next/app'
import Script from 'next/script'
// reusable gtag function
import * as gtag from 'config/gtag'
function MyApp({Component, pageProps}: AppProps) {
const router = useRouter()
useEffect(() => {
// function to get the current page url and pass it to gtag pageView() function
const handleRouteChange = (url: string) => {
gtag.pageView(url);
}
router.events.on('routeChangeComplete', handleRouteChange);
router.events.on('hashChangeComplete', handleRouteChange);
return () => {
router.events.off('routeChangeComplete', handleRouteChange);
router.events.off('hashChangeComplete', handleRouteChange);
}
}, [router.events])
return (
<>
{/* gtag script code */}
<Script
strategy="afterInteractive"
src={`https://www.googletagmanager.com/gtag/js?id=${gtag.GA_TRACKING_ID}`}
/>
<Script
id="gtag-init"
strategy="afterInteractive"
dangerouslySetInnerHTML={{
__html: `
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', '${gtag.GA_TRACKING_ID}', {
page_path: window.location.pathname
});`,
}}
/>
<Component {...pageProps} />
</>
)
}
Now, run your app and navigate to other pages within your application. Head over to your analytics dashboard. You should see some analytics being displayed such as the number of users on your site, the pages you have visited, etc.
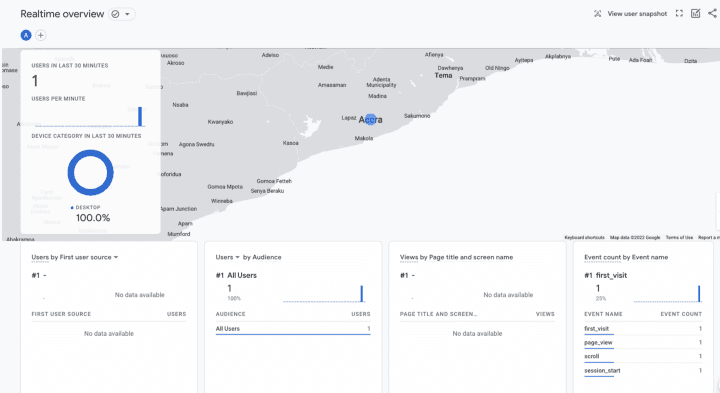
At this point, you have everything to start gathering and making informed decisions about the data being recorded on your website.
Part 2: Questions I needed answers to when setting up Google Analytics
During my research in setting up Analytics within my NextJs application, I came across similar terms such as Google Analytics, Google Analytics Tag, Google Analytics Tag 4, Firebase Analytics, Google Analytics for Firebase, etc.
I wanted to know the difference between each term. Hence, I started searching for answers to those questions.
What is the difference between Google Analytics, Firebase Analytics, Google Analytics 4, etc.
Google Analytics “classic” is a web analytics service offered by Google that tracks and reports website traffic. It was launched in the year 2005 after acquiring Urchin.
Google Analytics is in its fourth version. Below is the list of the previous versions:
- The first version of Google Analytics(GA1) is the Classic Google Analytics(ga.js JavascriptLibrary).
- The second version of Google Analytics(GA2) is the Universal Analytics(analytics.js Javascript Library).
- The third version of Google Analytics(GA3) is also Universal Analytics but it uses the gtag.js Javascript Library.
Note: Google Analytics 4(the fourth version of Google Analytics “classic”) also uses the gtag.js Javascript Library but uses a new measurement ID.
Firebase Analytics on the other hand is an analytics feature within the Firebase platform that allows data collection of mobile app interactions.
In 2014, Google acquired the independent company, Firebase, and up until 2021, the name Firebase Analytics was deprecated. Firebase Analytics is now known as Google Analytics(after being changed to Google Analytics for Firebase) and is still the only analytics module within the Firebase platform.
Honestly, if you’re confused as me about the differences in names, it is no fault of yours. Google has been poor when it comes to naming this product. Firebase Analytics, as explained above, was changed to Google Analytics for Firebase, then later to Google Analytics. And Google Analytics, as we already know before the Firebase acquisition, is now called Google Analytics 4.
Does it mean that Google Analytics for Firebase and Google Analytics(Google Analytics 4) have the same features?
Both Google Analytics for Firebase(aka Firebase Analytics) and Google Analytics 4 allow you to track events on both native/hybrid apps and websites/web apps.
So yes, both products currently have the same features. The only difference is the terminologies used in these environments. For example, what you think of as a Firebase project is generally known as property in Google Analytics 4 environment.
Thank you for getting to this point. If you have any suggestions or questions, kindly leave them in the comment section. Also, for any further reading, check out the resources listed below: